# 元素尺寸
# - offsetWidth/offsetHeight
offsetWidth\offsetHeight 属性是只读属性,它返回该元素的像素宽度与高度,包含内边距(padding)和边框(border)和滚动条,不包含外边距(margin),单位是像素 px。
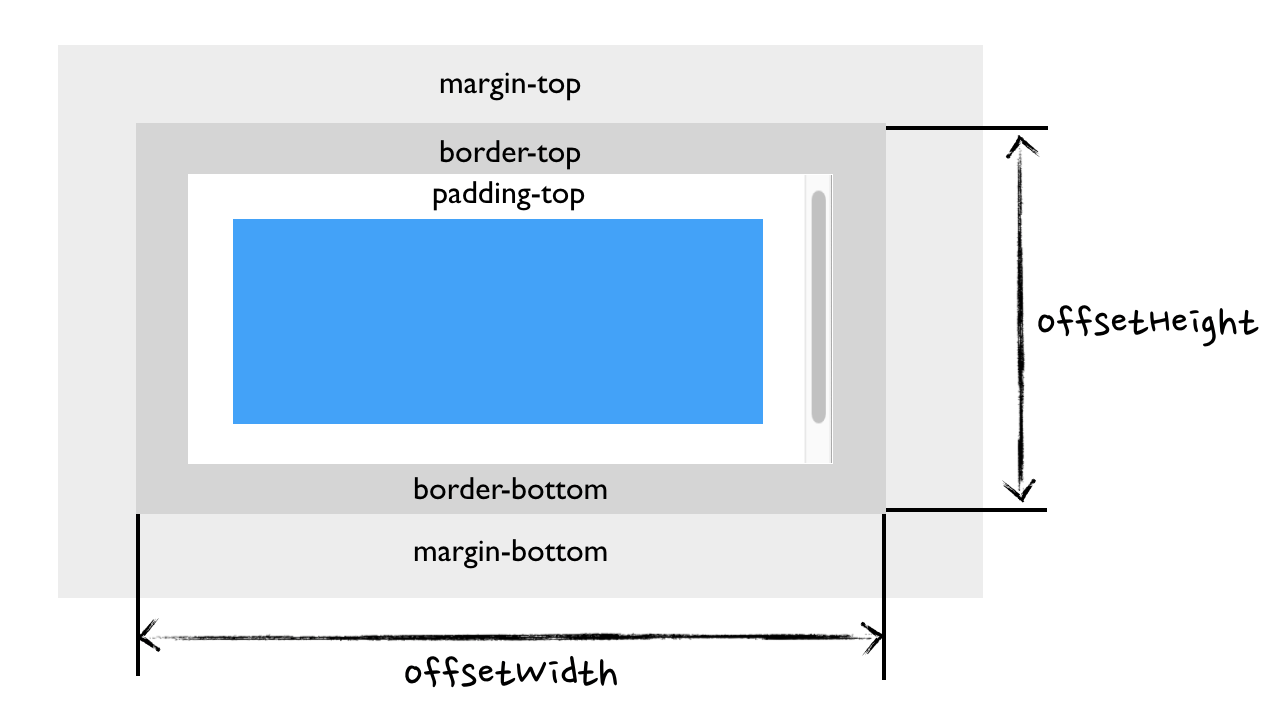
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
*{
padding:0;
margin:0;
}
#root{
width:500px;
height: 500px;
padding:20px;
border:30px solid yellow;
overflow: auto;
}
#app{
width: 800px;
height: 700px;
background: skyblue;
padding:20px;
border:10px solid green;
margin:20px;
}
</style>
</head>
<body>
<div id="root">
<div id="app"></div>
</div>
</body>
<script>
var app = document.querySelector("#app");
var root = document.querySelector("#root");
// offsetWidth/offsetHeight:是一个只读属性,该属性返回是元素的宽度/高度像素。
// offsetWidth包含:padding+width+border
// offsetHeight包含:padding+height+border
console.log("app->offsetWidth",app.offsetWidth);// 860
console.log("app->offsetHeight",app.offsetHeight);// 760
console.log("root-offsetWidth",root.offsetWidth);// 600
console.log("root-offsetHeight",root.offsetHeight);// 600
</script>
</html>
# - clientWidth/clientHeight
clientWidth\clientHeight属性是只读属性,它返回该元素的像素宽度与高度,包含内边距(padding)和边(border),不包含外边距(margin),单位是像素 px。

<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
*{
padding:0;
margin:0;
}
#root{
width:500px;
height: 500px;
padding:20px;
border:30px solid yellow;
overflow: auto;
}
#app{
width: 800px;
height: 700px;
background: skyblue;
padding:20px;
border:10px solid green;
margin:20px;
}
</style>
</head>
<body>
<div id="root">
<div id="app"></div>
</div>
</body>
<script>
// clientWidth与clientHeight也是获得元素的宽度与高度。
// 不包含边框border,margin,滚动条。
// clientWidth: width+padding
// clientHeight: height+padding
var app = document.querySelector("#app");
var root = document.querySelector("#root");
console.log("app->clientWidth",app.clientWidth);// 840
console.log("app->clientHeight",app.clientHeight);// 740
console.log("root->clientWidth",root.clientWidth);// 523
console.log("root->clientHeight",root.clientHeight);// 523
</script>
</html>
# - scrollWidth/scrollHeight
scrollWidth\ scrollHeight 属性是只读属性,它返回该元素的像素宽度与高度,包含内边距(padding),不包含外边距(margin)、边框(border),是一个整数,单位是像素 px。
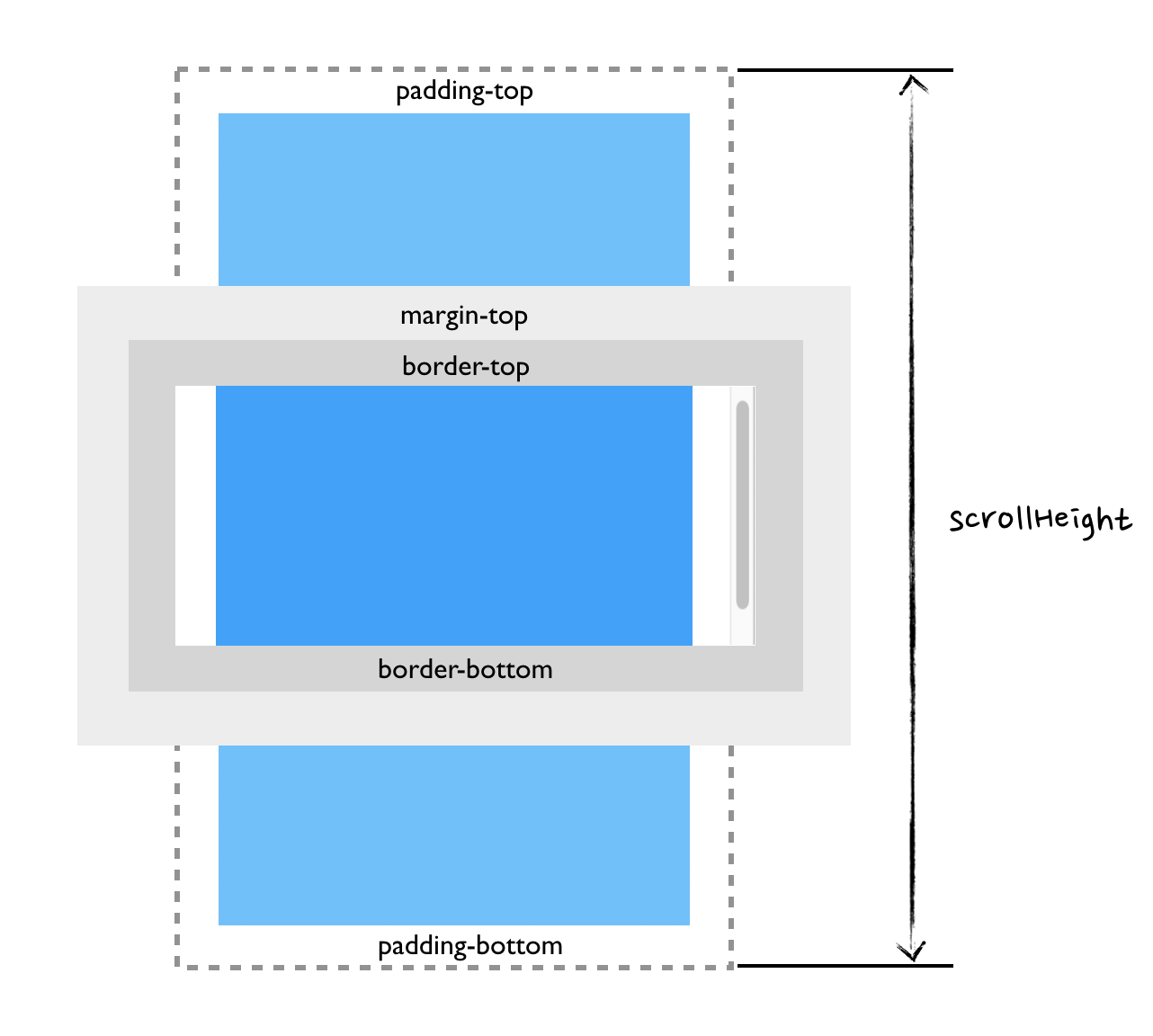
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
*{
padding:0;
margin:0;
}
#root{
width:500px;
height: 500px;
padding:20px;
border:30px solid yellow;
overflow: auto;
}
#app{
width: 800px;
height: 700px;
background: skyblue;
padding:20px;
border:10px solid green;
margin:20px;
}
</style>
</head>
<body>
<div id="root">
<div id="app"></div>
</div>
</body>
<script>
// scrollWdith/scrollHeight:在不使用滚动条的情况下,所包含的内容的宽度/高度。
// 理解:在不使用滚动条的情况下与clientWidth/clientHeight值相同。
// 前提:包含的内容不超过自身的情况下。
// 如果使用滚动条:自身的padding+包裹元素的margin+包裹元素的border+包裹元素的padding+包裹元素的width/height
var app = document.querySelector("#app");
var root = document.querySelector("#root");
// console.log("app->scrollWidth",app.scrollWidth);
// console.log("app->clientWith",app.clientWidth);
// console.log("app->scrollHeight",app.scrollHeight);
// console.log("app->clientHeight值相同",app.clientHeight);
console.log("root->scrollWidth",root.scrollWidth);
console.log("root->clientWith",root.clientWidth);
console.log("root->scrollHeight",root.scrollHeight);
console.log("root->clientHeight值相同",root.clientHeight);
</script>
</html>
# - clientLeft/clientTop
clientLeft 表示一个元素的左边框的宽度,以像素表示。
clientLeft 表示一个元素的上边框的宽度,以像素表示。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
*{
padding:0;
margin:0;
}
#root{
width:500px;
height: 500px;
padding:20px;
border-left:20px solid yellow;
border-top:30px solid green;
overflow: auto;
}
#app{
width: 800px;
height: 700px;
background: skyblue;
padding:20px;
border:10px solid green;
margin:20px;
}
</style>
</head>
<body>
<div id="root">
<div id="app"></div>
</div>
</body>
<script>
// clientLeft/clientTop:左边框宽度/上边框宽度
var app = document.querySelector("#app");
var root = document.querySelector("#root");
console.log(app.clientLeft);
console.log(app.clientTop);
console.log(root.clientLeft);
console.log(root.clientTop);
</script>
</html>
# - 视口宽度
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div style="height:1000px;"></div>
</body>
<script>
console.log("获得浏览器当中最纯净的部分,不包含浏览器边框+不包含滚动条",document.documentElement.clientWidth);
console.log("纯净部分+滚动条",window.innerWidth);
console.log("纯净部分+滚动条+浏览器边框",window.outerWidth);
console.log("显示器的显示分辨率",screen.width);// 1366
</script>
</html>
# - 案例:触壁反弹
效果
代码
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>案例-触壁反弹-前端猫</title> <style> *{ padding:0; margin:0; } #root{ position: relative; width: 500px; height: 500px; border:20px solid skyblue; margin:0 auto; } #ball{ position: absolute; width: 50px; height: 50px; padding:10px; border:2px solid green; border-radius: 100px; background-image: url("../../../icon.png"); background-repeat: no-repeat; background-position: center; } </style> </head> <body> <div id="root"> <div id="ball"></div> </div> </body> <script> var root = document.getElementById("root"); var ball = document.getElementById("ball"); var x = 0;// left值 横向的距离 var y = 0;// top值 纵向的距离 // left最大值 var maxLeft = root.clientWidth - ball.offsetWidth; // top最大值 var maxTop = root.clientHeight - ball.offsetHeight; // left移动速度,正数向右,负数向左 var speedX = 5; // top移动速度,正数向下,负数向上 var speedY = 3; setInterval(function (){ x+=speedX; y+=speedY; // 横向 if(x>maxLeft){// 限制最大值 x=maxLeft; speedX = -speedX; }else if(x<0){// 限制最小值 x=0; speedX = -speedX; } // 纵向 if(y>maxTop){// 限制最大值 y=maxTop; speedY = -speedY; }else if(y<0){// 限制最小值 y=0; speedY = -speedY; } ball.style.left = x+"px"; ball.style.top = y+"px"; },30) </script> </html>
# - 案例:多个小球躁动
效果
代码
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>案例:多个小球-前端猫</title> <style> * { padding: 0; margin: 0; } #root { position: relative; width: 500px; height: 500px; border: 20px solid skyblue; margin: 0 auto; } </style> </head> <body> <div id="root"> </div> </body> <script> var root = document.querySelector("#root"); var rootClientW = root.clientWidth; var rootClientH = root.clientHeight; // 小球数量 var ballNum = 30; function getRandom(min, max) { console.log(min, max); return Math.floor(Math.random() * (max - min + 1) + min); } var str = ""; for (var i = 0; i < ballNum; i++) { var wh = getRandom(20, 100); var topX = getRandom(0, rootClientH - wh); var left = getRandom(0, rootClientW - wh); var ball = (` <div data-speedX="${getRandom(3, 20)}" data-speedY="${getRandom(3, 20)}" style="top:${topX}px;left:${left}px; position: absolute;width:${wh}px; height:${wh}px;border-radius:100%; background:rgb(${getRandom(1, 255)},${getRandom(1, 255)},${getRandom(1, 255)})"> </div> `); str += ball; } root.innerHTML = str; var balls = document.querySelectorAll("#root div"); setInterval(function () { balls.forEach(function (item) { // item.style.left =200 var x = item.style.left.replace("px", "") / 1;// 移除px/1 var y = item.style.top.replace("px", "") / 1;// 移除px/1 var maxLeft = root.clientWidth - item.offsetWidth; var maxTop = root.clientHeight - item.offsetHeight; var speedX = item.dataset.speedx / 1; var speedY = item.dataset.speedy / 1; console.log(speedX, speedY) x += speedX; y += speedY; // 横向 if (x > maxLeft) {// 限制最大值 x = maxLeft; item.dataset.speedx = -speedX; } else if (x < 0) {// 限制最小值 x = 0; item.dataset.speedx = -speedX; } // 纵向 if (y > maxTop) {// 限制最大值 y = maxTop; item.dataset.speedy = -speedY; } else if (y < 0) {// 限制最小值 y = 0; item.dataset.speedy = -speedY; } item.style.left = x + "px"; item.style.top = y + "px"; }) }, 30) </script> </html>